WordPress で Jamstack
by Yuji Arai
はじめに
Next.js + WordPress API で Jamstack を構築してみようかと思います。
便利なヘッドレスCMSはたくさんありますが、プランによってはお金がかかることが多いので。
WordPressのAPI化
まずはWordPressのAPI化です。
WordPressはデフォルトでも特定のURLを叩くことでJSON形式で値を取得することはできるかと思いますが、それだと不要なデータが多くあったりするためfunctions.phpに独自で実装しました。
以下、手順になります。
①空のテーマを作る
wp-content/themes/
上記ののディレクトリ配下にテーマ用の適当なディレクトリを作成し3つの空のファイルを格納します。
- functions.php
- index.php(今回は触らない)
- style.css(今回は触らない)
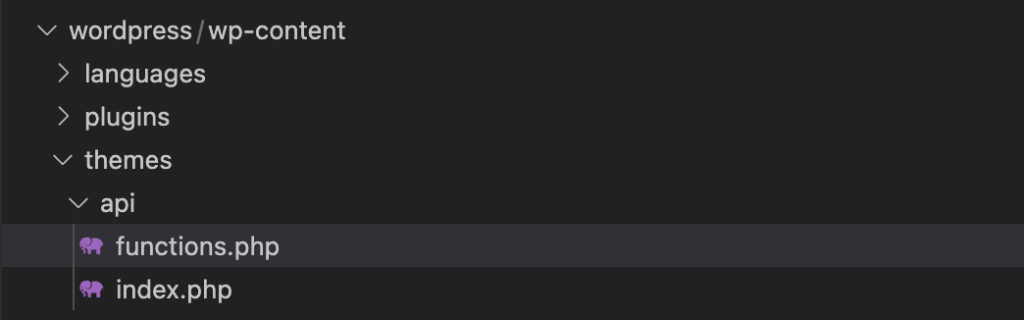
②functions.phpに記事取得の処理を書く
ファイルを格納できたらfunctions.phpに記事取得の処理を書きます。
今回は以下の記事を参考にさせていただきました。
https://designsupply-web.com/media/development/6838/
以下はfunctions.phpのコードになります。以下のコードで投稿の一覧と、特定の投稿を取得することができます(スラッグ指定)。一応簡易的にAPI KEYによる認証もしています。
これでAPIの準備は完了です。
③JSONを取得できるかテストしてみる
以下のURLでそれぞれ値を取得できるはずです。
httpヘッダにAPIキーを含めるのも忘れずにお願いします。
一覧:http://localhost/wp-json/wp/api/blog/
詳細:http://localhost/wp-json/wp/api/blog/{記事のスラッグ}
※localhost部分は環境によって適宜変更してください。
一覧の取得結果
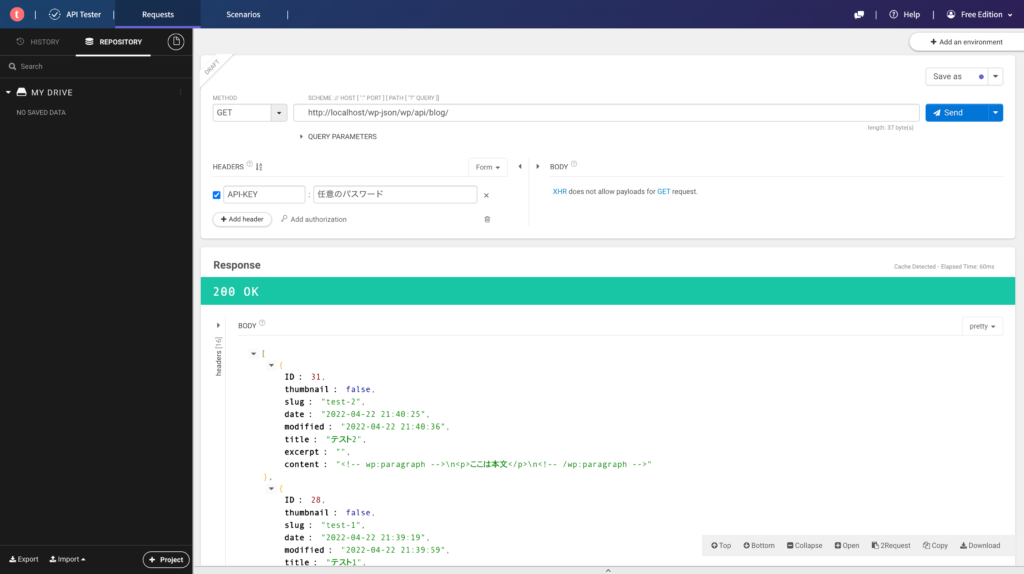
詳細の取得結果
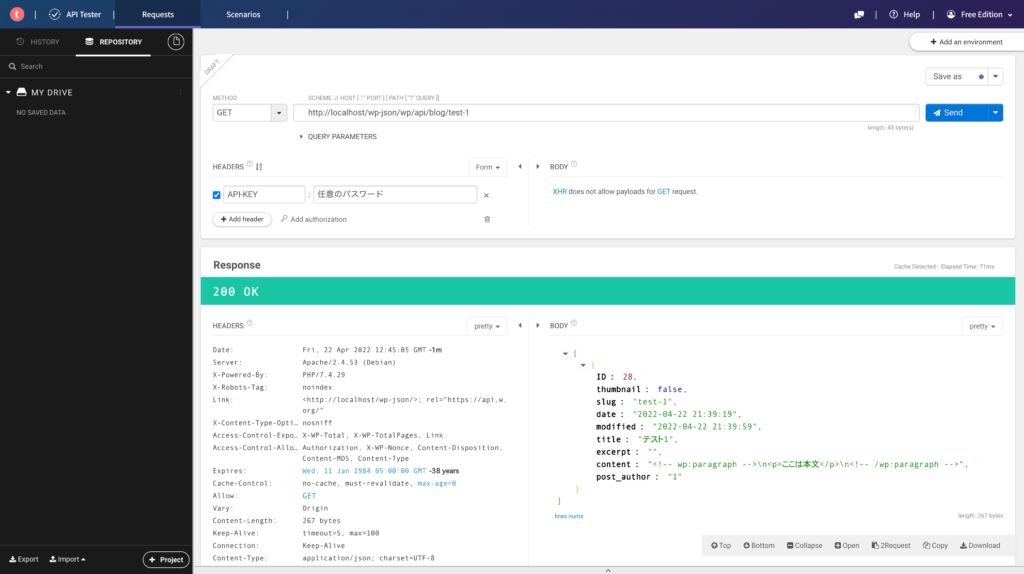
これでAPIはひとまず完成です。
Next.jsの実装
APIをコールしたい箇所で getStaticProps を利用して値を取得します。
以下は一覧の例です。
詳細は getStaticPath で一覧取得のAPIをコールし、getStaticProps で詳細情報を取得します。
これで必要なデータは取得できるかと思います。
GitHub Actionsで記事投稿をしたときに自動で反映されるようにする
現状の実装だけでは記事を投稿・編集しても本番のサーバーに反映されることはないので、GitHub Actionsを利用してNext.jsのビルドからFTPで本番のサーバーにデータを転送するようにします。
まずはGitHubにログインしてNext.jsのソースをアップロードしているリポジトリに移動します。
Actions タブに移動し New workflow ボタンを押すことで新しく workflow を作成することができます。以下のyamlを記述してください。
server、username、password、server-dirは任意の値を渡すようにしてください。直接記述するのではなく、Settings の Secrets を利用するようにしてください。
server-dirはライブラリの仕様上最後に/をつけるようにしてください。
上記のyamlではmasterブランチのソースを更新することでも自動的にビルドとFTPを用いたアップロードが行われます。
不要であれば以下の部分を削除してください。
記事投稿後にGitHub Actionsを実行するようにする
まずはGitHub Actionsを外部から実行できるようにします。外部から実行するためにはPersonal access tokenが必要なので、GitHubページから作成します。
Personal access tokenの設定
GitHub個人のSettingsページから
Developer settings > Personal access tokens
に移動します。
Noteは任意のものを入力し有効期限の入力とworkflowにチェックを入れてトークンを生成します。
これで外部からGitHub Actionsを実行できるようになります。
functions.phpに追記
あとは以下のソースをfunctions.phpに追記するだけです。
記事投稿したタイミングでGitHub Actionsを実行するようにします。
URLとAuthorizationのトークンは自身のものに書き換えてください。
こちらで一通りの実装は完了です。
終わりに
まだかなり簡易的な実装ではありますが一通り動くようになったかと思います。
こちらをベースに色々と改変を加えていければと思います。